Subroutine-function
A function is a subroutine that returns a result (number, character string, etc.). div>
Imagine that you have ordered a product from an online store. From a programming point of view, you called some subroutine, and unlike a procedure, this subroutine must return a result - deliver the product you ordered. These subroutines are called functions.
A function is formatted in exactly the same way as a procedure. The only difference from the procedure is the presence of a special operator return, after which the value to be returned to the main program is written.
A function that returns the arithmetic mean of two integers would look like this:
def average(a, b):
avg = (a + b) / 2
return avg
It remains to understand how to call this function in the main program:
You should not call a function in the same way as a procedure:
average(10, 5)
The value returned by the function will be lost. It is as if the goods from the online store were not given to anyone, but thrown away. It is unlikely that the customer will like it.
It's more correct to save the result in a variable (or print it to the screen):
a = average(10, 5)
print(average(10, 5))
Need to remember!
- A function subroutine is formatted in the same way as a procedure.
- The function is called at the place in the program where you would put the value. For example, as a parameter of a print() statement or in an arithmetic expression.
|
Euclid's algorithm
Euclid's algorithm — efficient BC" title="Algorithm">Algorithm To Find Greatest Common Divisor two Integers (or general measures two Lines). The algorithm is named after Greek Math Euclid (3rd century B.C. ), who first described it in VII and X books « Beginnings". It is one of the oldest numerical algorithms in use today.
Remember the math.
Greatest common divisor of two natural numbers (gcd) is the largest natural number by which they are divisible.
For example, the numbers 12 and 18 have common divisors: 2, 3, 6. The greatest common divisor is 6. This is written as: gcd(12, 18) = 6
In programming, there are several implementations of the Euclid algorithm. Here is a description of one of them in the form of a block diagram.
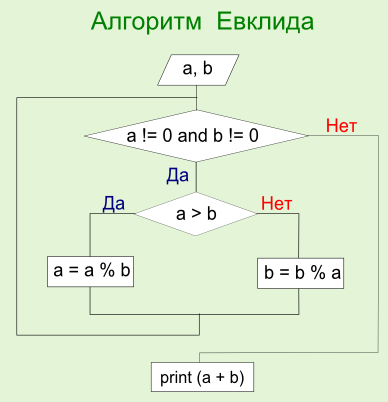
Try to implement this algorithm.
|
Logic functions
Often programmers use boolean functions that return boolean values "true" or "false" ( trueor false)
Such functions are useful for check some property.
Let's consider two examples of writing a logical function that checks if a number is even.
Description |
Subroutine example |
1) Better way: the result of the expression n % 2 == 0 will be true (True) or false (False) |
def isEven(n):
return (n % 2 == 0)
|
2) You can write it like that, but it’s better not to do a longer record anyway |
def isEven(n):
if n % 2 == 0:
return true;
else:
return False
|
And the last note about working with functions and procedures: the number of functions and procedures in the program is not limited. In addition, one subroutine can call another subroutine and even itself.
|