Problem description | | Progress |
Темы:
Computer science
Write a program that calculates the value of an expression using a known formula.
\({x + y\over {x +1}}-{x\cdot y-12 \over 34 + x}\)
x and y are integer type variables, entered from the keyboard.
The program should output one number - the result of the expression evaluation.
Hint: don't forget to get a real number when dividing!
| |
|
Темы:
Computer science
Write a program that calculates the value of the expression
\({x + y\over {x +1}}-{x\cdot y-12 \over 34 + x}\)
Input
x and y are integer variables.
Output
output a single number - the value of the expression
Examples
№ |
Input |
Output |
1 |
1 2 |
1.786 |
Hint
Do not forget that when dividing, you need to get a real number!
| |
|
Темы:
Computer science
Write a program that determines the distance between two points with given coordinates x1 and x2 code> on the numeric axis. The distance between two points is calculated using the formula \(|x_2 - x_1|\).
The first line of input contains two real numbers. Output one real number – distance between two points. Round your answer to 3 decimal places.
Examples
# |
Input |
Output |
1 |
100000 0 |
100000.000 |
| |
|
Темы:
Computer science
Write a program that determines the distance between two points given coordinates x1 and x2 on the number line. The distance between two points is calculated using the formula |x2 − x1|.
The first input line contains a real number x1, the second line contains a real number x2. Output one real number – distance between two points.
Sample input and output data.
| |
|
Темы:
Computer science
Write a program that calculates the value of an expression using a known formula:
\({x + y\over {x +1}}-{x\cdot y-12 \over 34 + x}\)
x and y are variables of integer type, entered from the keyboard.
The program should output one number - the result of the expression evaluation.
Hint: do not forget that when dividing, you need to get a real number!
Sample input and output data.
Input |
Output |
1
2 |
1.78571428571429 |
| |
|
Темы:
Computer science
Whole numbers
Prince is very fond of math. For his research, he needs to learn to quickly find out if one of the two numbers is divisible by the other. Help Prince write a program so he can get an answer instantly.
Input format
Given two natural numbers, each not more than 100.
Output format
The program should output the number 1 if one of the numbers is divisible by another. Otherwise, you must output any other number not equal to 1 , and not exceeding 109.
Examples
N |
Input |
Output |
1 |
2 4 |
1 |
2 |
4 2 |
1 |
3 |
2 5 |
500 |
| |
|
Темы:
Computer science
Write a program that determines the distance between two points with the given coordinates x1 and x2 on the numerical axis. The distance between two points is calculated using the formula
|x2 - x1|
The first line of input contains two real numbers. Output one real number - the distance between two points.
Example
N |
Input |
Output |
1 |
100000 0 |
100000.000000 |
| |
|
Темы:
Computer science
Given a checkered field N x M, all cells of the field are initially white. The machine can:
paint cell (i, j) black.
for cell (i,j) to find out its nearest white neighbors vertically and horizontally.
Given a sequence of commands for the machine. It is required to execute these commands in the specified sequence, and for each command to query the nearest white neighbors, indicate the result of its execution.
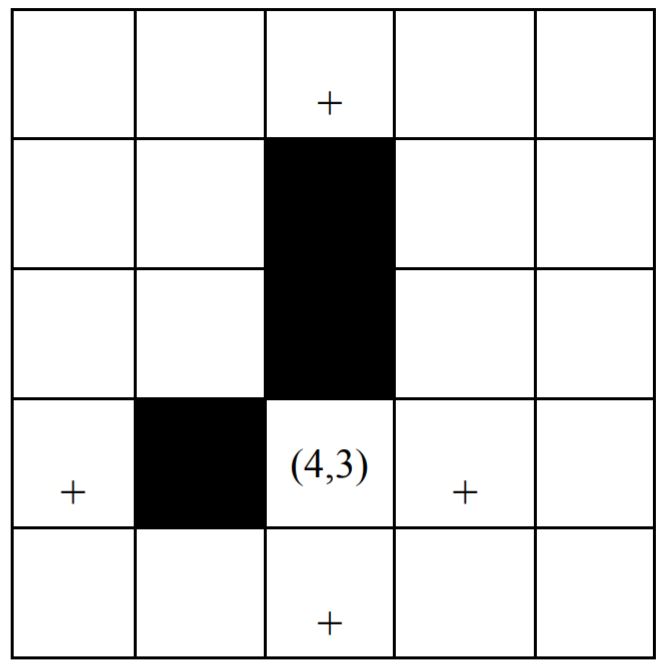
Input
First enter the field sizes N and M (1 ≤ N ≤ 20, 1 ≤ M ≤ 50000), then the number of commands K (1 ≤ K ≤ 105), and then themselves commands. Commands are written one per line in the following format:
Color i j — coloring cell (i, j) black;
Neighbors i j — finding white neighbors for a WHITE cell (i,j).
1≤ i≤ N, 1 ≤ j ≤ M.
Imprint
For each Neighbors query, you first need to display the number of nearest white neighbors (or 0 if there are no white cells left on either side), and then their coordinates (neighbors can be listed in any order). If there are no Neighbors requests, nothing should be output.
Examples
# |
Input |
Output |
1 |
5 |
0 1 2 3 4
1 0 1 2 3
2 1 0 1 2
3 2 1 0 1
4 3 2 1 0
|
| |
|
Темы:
Number systems
Computer science
Input
The program receives a natural number as input - N .
Imprint
Display the representation of a number in hexadecimal notation, in which the digits of the number are written in reverse order, that is, from the end. To represent numbers from 10 to 15, use capital English letters from 'A ' to 'F '.
Examples
| |
|
Темы:
Number systems
Computer science
Write a program that converts the number N from base r to decimal.
Input
The program receives two natural numbers as input: N and r (2 <= r <= 9). It is guaranteed that the number N is the correct representation of the number in the number system with the base r (that is, it contains the numbers from 0 to r- 1 ).
Imprint
Display the number in decimal notation.
Examples
| |
|
ID 39929.
2(6)
Темы:
Computer science
The following ciphered radiogram was received from the intelligence officer, transmitted using Morse code.
• – – – • • – – • • • •  ;• – – •
During the transmission of the radiogram, the division into letters was lost, but it is known that only the following letters were used in the radiogram.
M |
N |
C |
U |
A |
E |
– – |
– • |
• • • |
• • – |
• – |
– – – |
Define the text of the radiogram. In your answer, write down the resulting word (a set of letters).
| |
|
Темы:
Computer science
Complete the program to output a "- " (a sign minus) if the number entered from the keyboard is negative, and a "+ " (sign plus) otherwise.
| |
|